szukam pluginu na JB, ponieważ chciałbym wprowadzić inny model dla więźniów. robi mi już kolega z adresem forum na plecach itp, ale mniejsza. jest mi potrzebny plugin pod to.
Witamy w Nieoficjalnym polskim support'cie AMX Mod X
Witamy w Nieoficjalnym polskim support'cie AMX Mod X, jak w większości społeczności internetowych musisz się zarejestrować aby móc odpowiadać lub zakładać nowe tematy, ale nie bój się to jest prosty proces w którym wymagamy minimalnych informacji.
|
Guest Message by DevFuse
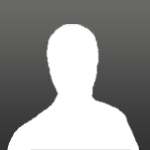
Dowolny model
Temat rozp. tNt, 14.12.2009 15:21
1 odpowiedź w tym temacie
#1
Napisano 14.12.2009 15:21
#2
Napisano 14.12.2009 15:33
#include <amxmodx> #include <fakemeta> #include <hamsandwich> #include <cstrike> new const ZOMBIE_MODEL[] = 'modelwiz" // The model we're gonna use for zombies #define MODELSET_TASK 100 // an offset for our models task #define MODELCHANGE_DELAY 0.5 // delay between model changes new Float:g_models_targettime // target time for the last model change new Float:g_roundstarttime // last round start time new g_has_custom_model[33] // whether the player is using a custom model new g_player_model[33][32] // player's model name (string) new g_zombie[33] // whether the player is a zombie /*================================================================================ [Plugin Start] =================================================================================*/ public plugin_precache() { new modelpath[100] formatex( modelpath, charsmax( modelpath ), "models/player/%s/%s.mdl", ZOMBIE_MODEL, ZOMBIE_MODEL ) engfunc( EngFunc_PrecacheModel, modelpath ) } public plugin_init() { register_plugin( "Player Model Changer Example", "0.3", "MeRcyLeZZ" ) register_event( "HLTV", "event_round_start", "a", "1=0", "2=0" ) RegisterHam( Ham_Spawn, "player", "fw_PlayerSpawn", 1 ) register_forward( FM_SetClientKeyValue, "fw_SetClientKeyValue" ) register_forward( FM_ClientUserInfoChanged, "fw_ClientUserInfoChanged" ) } /*================================================================================ [Round Start Event] =================================================================================*/ public event_round_start() { g_roundstarttime = get_gametime() } /*================================================================================ [Player Spawn Event] =================================================================================*/ public fw_PlayerSpawn( id ) { // Not alive or didn't join a team yet if ( !is_user_alive( id ) || !cs_get_user_team( id ) ) return; // Set to zombie if on Terrorist team g_zombie[id] = cs_get_user_team( id ) == CS_TEAM_T ? true : false; // Remove previous tasks (if any) remove_task( id + MODELSET_TASK ) // Check whether the player is a zombie if ( g_zombie[id] ) { // Store our custom model in g_player_model[id] copy( g_player_model[id], charsmax( g_player_model[] ), ZOMBIE_MODEL ) // Get current model new currentmodel[32] fm_get_user_model( id, currentmodel, charsmax( currentmodel ) ) // Check whether it matches the custom model if ( !equal( currentmodel, g_player_model[id] ) ) { // An additional delay is offset at round start // since SVC_BAD is more likely to be triggered there if ( get_gametime() - g_roundstarttime < 5.0 ) set_task( 5.0 * MODELCHANGE_DELAY, "fm_user_model_update", id + MODELSET_TASK ) else fm_user_model_update( id + MODELSET_TASK ) } } // Not a zombie, but still has a custom model else if ( g_has_custom_model[id] ) { // Reset it back to the default one fm_reset_user_model( id ) } } /*================================================================================ [Forwards] =================================================================================*/ public fw_SetClientKeyValue( id, const infobuffer[], const key[] ) { // Block CS model changes if ( g_has_custom_model[id] && equal( key, "model" ) ) return FMRES_SUPERCEDE; return FMRES_IGNORED; } public fw_ClientUserInfoChanged( id ) { // Player doesn't have a custom model if ( !g_has_custom_model[id] ) return FMRES_IGNORED; // Get current model static currentmodel[32] fm_get_user_model( id, currentmodel, charsmax( currentmodel ) ) // Check whether it matches the custom model - if not, set it again if ( !equal( currentmodel, g_player_model[id] ) && !task_exists( id + MODELSET_TASK ) ) fm_set_user_model( id + MODELSET_TASK ) return FMRES_IGNORED; } /*================================================================================ [Tasks] =================================================================================*/ public fm_user_model_update( taskid ) { static Float:current_time current_time = get_gametime() // Do we need a delay? if ( current_time - g_models_targettime >= MODELCHANGE_DELAY ) { fm_set_user_model( taskid ) g_models_targettime = current_time } else { set_task( (g_models_targettime + MODELCHANGE_DELAY) - current_time, "fm_set_user_model", taskid ) g_models_targettime = g_models_targettime + MODELCHANGE_DELAY } } public fm_set_user_model( player ) { // Get actual player id player -= MODELSET_TASK // Set new model engfunc( EngFunc_SetClientKeyValue, player, engfunc( EngFunc_GetInfoKeyBuffer, player ), "model", g_player_model[player] ) // Remember this player has a custom model g_has_custom_model[player] = true } /*================================================================================ [Stocks] =================================================================================*/ stock fm_get_user_model( player, model[], len ) { // Retrieve current model engfunc( EngFunc_InfoKeyValue, engfunc( EngFunc_GetInfoKeyBuffer, player ), "model", model, len ) } stock fm_reset_user_model( player ) { // Player doesn't have a custom model any longer g_has_custom_model[player] = false dllfunc( DLLFunc_ClientUserInfoChanged, player, engfunc( EngFunc_GetInfoKeyBuffer, player ) ) }
Kompilujesz. W zmiennej:
new const ZOMBIE_MODEL[] = 'modelwiz" // The model we're gonna use for zombiesustalasz nazwe pliku i ścieżkę!
np dasz model2 to ten model musisz wkleić do
cstrike/models/player/model2/model2.mdl ,a jeżeli nic nie zmieniasz: cstrike/models/player/modelwiz/modelwiz.mdl
Plugin nie powoduje SVC_BAD nawet przy 20 osobach.

Nigdy mnie nie zawiódł.
Użytkownicy przeglądający ten temat: 1
0 użytkowników, 1 gości, 0 anonimowych